an expression which returns the newly
assigned value. Some programmers will use that feature to write things like the following.
y = (x = 2 * x);// double x, and also put x's new value in y
Truncation
The opposite of promotion, truncation moves a value from a type to a smaller type. In
that case, the compiler just drops the extra bits. It may or may not generate a compile
time warning of the loss of information. Assigning from an integer to a smaller integer
(e.g.. long to int, or int to char) drops the most significant bits. Assigning from a
floating point type to an integer drops the fractional part of the number.
char ch;
int i;
i = 321;
ch = i;// truncation of an int value to fit in a char
// ch is now 65
The assignment will drop the upper bits of the int 321. The lower 8 bits of the number
321 represents the number 65 (321 - 256). So the value of ch will be (char)65 which
happens to be 'A'.
The assignment of a floating point type to an integer type will drop the fractional part of
the number. The following code will set i to the value 3. This happens when assigning a
floating point number to an integer or passing a floating point number to a function which
takes an integer.
double pi;
int i;
pi = 3.14159;
i = pi;// truncation of a double to fit in an int
// i is now 3
Pitfall -- int vs. float Arithmetic
Here's an example of the sort of code where int vs. float arithmetic can cause
problems. Suppose the following code is supposed to scale a homework score in the
range 0..20 to be in the range 0..100.
{
int score;
...// suppose score gets set in the range 0..20 somehow
7
score = (score / 20) * 100;// NO -- score/20 truncates to 0
...
Unfortunately, score will almost always be set to 0 for this code because the integer
division in the expression (score/20) will be 0 for every value of score less than 20.
The fix is to force the quotient to be computed as a floating point number...
score = ((double)score / 20) * 100;// OK -- floating point division from cast
score = (score / 20.0) * 100;// OK -- floating point division from 20.0
score = (int)(score / 20.0) * 100;// NO -- the (int) truncates the floating
// quotient back to 0
No Boolean -- Use int
C does not have a distinct boolean type-- int is used instead. The language treats integer
0 as false and all non-zero values as true. So the statement...
i = 0;
while (i - 10) {
...
will execute until the variable i takes on the value 10 at which time the expression (i -
10) will become false (i.e. 0). (we'll see the while() statement a bit later)
Mathematical Operators
C includes the usual binary and unary arithmetic operators. See the appendix for the table
of precedence. Personally, I just use parenthesis liberally to avoid any bugs due to a
misunderstanding of precedence. The operators are sensitive to the type of the operands.
So division (/) with two integer arguments will do integer division. If either argument is
a float, it does floating point division. So (6/4) evaluates to 1 while (6/4.0)
evaluates to 1.5 -- the 6 is promoted to 6.0 before the division.
+Addition
-Subtraction
/Division
*Multiplication
%Remainder (mod)
Unary Increment Operators: ++ --
The unary ++ and -- operators increment or decrement the value in a variable. There are
"pre" and "post" variants for both operators which do slightly different things (explained
below)
var++increment"post" variant
++varincrement"pre" variant
8
var--decrement"post" variant
--vardecrement"pre" variant
int i = 42;
i++;// increment on i
// i is now 43
i--;// decrement on i
// i is now 42
Pre and Post Variations
The Pre/Post variation has to do with nesting a variable with the increment or decrement
operator inside an expression -- should the entire expression represent the value of the
variable before or after the change? I never use the operators in this way (see below), but
an example looks like...
int i = 42;
int j;
j = (i++ + 10);
// i is now 43
// j is now 52 (NOT 53)
j = (++i + 10)
// i is now 44
// j is now 54
C Programming Cleverness and Ego Issues
Relying on the difference between the pre and post variations of these operators is a
classic area of C programmer ego showmanship. The syntax is a little tricky. It makes the
code a little shorter. These qualities drive some C programmers to show off how clever
they are. C invites this sort of thing since the language has many areas (this is just one
example) where the programmer can get a complex effect using a code which is short and
dense.
If I want j to depend on i's value before the increment, I write...
j = (i + 10);
i++;
Or if I want to j to use the value after the increment, I write...
i++;
j = (i + 10);
Now then, isn't that nicer? (editorial) Build programs that do something cool rather than
programs which flex the language's syntax. Syntax -- who cares?
Relational Operators
These operate on integer or floating
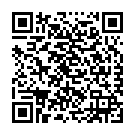
Continue reading on your phone by scaning this QR Code
Tip: The current page has been bookmarked automatically. If you wish to continue reading later, just open the
Dertz Homepage, and click on the 'continue reading' link at the bottom of the page.