always
convert from a type to compatible, larger type to avoid losing information.
Pitfall -- int Overflow
I once had a piece of code which tried to compute the number of bytes in a buffer with
the expression (k * 1024) where k was an int representing the number of kilobytes
I wanted. Unfortunately this was on a machine where int happened to be 16 bits. Since
k and 1024 were both int, there was no promotion. For values of k >= 32, the product
was too big to fit in the 16 bit int resulting in an overflow. The compiler can do
whatever it wants in overflow situations -- typically the high order bits just vanish. One
way to fix the code was to rewrite it as (k * 1024L) -- the long constant forced the
promotion of the int. This was not a fun bug to track down -- the expression sure looked
reasonable in the source code. Only stepping past the key line in the debugger showed the
overflow problem. "Professional Programmer's Language." This example also
demonstrates the way that C only promotes based on the types in an expression. The
compiler does not consider the values 32 or 1024 to realize that the operation will
overflow (in general, the values don't exist until run time anyway). The compiler just
looks at the compile time types, int and int in this case, and thinks everything is fine.
Floating point Types
floatSingle precision floating point numbertypical size: 32 bits
doubleDouble precision floating point numbertypical size: 64 bits
long doublePossibly even bigger floating point number (somewhat obscure)
Constants in the source code such as 3.14 default to type double unless the are suffixed
with an 'f' (float) or 'l' (long double). Single precision equates to about 6 digits of
5
precision and double is about 15 digits of precision. Most C programs use double for
their computations. The main reason to use float is to save memory if many numbers
need to be stored. The main thing to remember about floating point numbers is that they
are inexact. For example, what is the value of the following double expression?
(1.0/3.0 + 1.0/3.0 + 1.0/3.0)// is this equal to 1.0 exactly?
The sum may or may not be 1.0 exactly, and it may vary from one type of machine to
another. For this reason, you should never compare floating numbers to eachother for
equality (==) -- use inequality (<) comparisons instead. Realize that a correct C program
run on different computers may produce slightly different outputs in the rightmost digits
of its floating point computations.
Comments
Comments in C are enclosed by slash/star pairs: /* .. comments .. */ which
may cross multiple lines. C++ introduced a form of comment started by two slashes and
extending to the end of the line: // comment until the line end
The // comment form is so handy that many C compilers now also support it, although it
is not technically part of the C language.
Along with well-chosen function names, comments are an important part of well written
code. Comments should not just repeat what the code says. Comments should describe
what the code accomplishes which is much more interesting than a translation of what
each statement does. Comments should also narrate what is tricky or non-obvious about a
section of code.
Variables
As in most languages, a variable declaration reserves and names an area in memory at run
time to hold a value of particular type. Syntactically, C puts the type first followed by the
name of the variable. The following declares an int variable named "num" and the 2nd
line stores the value 42 into num.
int num;
num = 42;num42A variable corresponds to an area of memory which can store a value of the given type.
Making a drawing is an excellent way to think about the variables in a program. Draw
each variable as box with the current value inside the box. This may seem like a
"beginner" technique, but when I'm buried in some horribly complex programming
problem, I invariably resort to making a drawing to help think the problem through.
Variables, such as num, do not have their memory cleared or set in any way when they
are allocated at run time. Variables start with random values, and it is up to the program
to set them to something sensible before depending on their values.
Names in C are case sensitive so "x" and "X" refer to different variables. Names can
contain digits and underscores (_), but may not begin with a digit. Multiple variables can
be declared after the type by separating them with commas. C is a classical "compile
time" language -- the names of the variables, their types, and their implementations are all
flushed out by the compiler at compile time (as opposed to figuring such details out at run
time like an interpreter).
6
float x, y, z, X;
Assignment Operator =
The assignment operator is the single equals sign (=).
i = 6;
i = i + 1;
The assignment operator copies the value from its right hand side to the variable on its
left hand side. The assignment also acts as
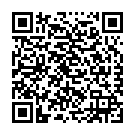
Continue reading on your phone by scaning this QR Code
Tip: The current page has been bookmarked automatically. If you wish to continue reading later, just open the
Dertz Homepage, and click on the 'continue reading' link at the bottom of the page.