point values and return a 0 or 1 boolean value.
==Equal
9
!=Not Equal
>Greater Than
>=Greater or Equal
<=Less or Equal
To see if x equals three, write something like:
if (x == 3) ...
Pitfall = ==
An absolutely classic pitfall is to write assignment (=) when you mean comparison (==).
This would not be such a problem, except the incorrect assignment version compiles fine
because the compiler assumes you mean to use the value returned by the assignment. This
is rarely what you want
if (x = 3) ...
This does not test if x is 3. This sets x to the value 3, and then returns the 3 to the if for
testing. 3 is not 0, so it counts as "true" every time. This is probably the single most
common error made by beginning C programmers. The problem is that the compiler is no
help -- it thinks both forms are fine, so the only defense is extreme vigilance when
coding. Or write "= ¹ ==" in big letters on the back of your hand before coding. This
mistake is an absolute classic and it's a bear to debug. Watch Out! And need I say:
"Professional Programmer's Language."
Logical Operators
The value 0 is false, anything else is true. The operators evaluate left to right and stop as
soon as the truth or falsity of the expression can be deduced. (Such operators are called
"short circuiting") In ANSI C, these are furthermore guaranteed to use 1 to represent true,
and not just some random non-zero bit pattern. However, there are many C programs out
there which use values other than 1 for true (non-zero pointers for example), so when
programming, do not assume that a true boolean is necessarily 1 exactly.
!Boolean not (unary)
&&Boolean and
||Boolean or
Bitwise Operators
C includes operators to manipulate memory at the bit level. This is useful for writing low-
level hardware or operating system code where the ordinary abstractions of numbers,
characters, pointers, etc... are insufficient -- an increasingly rare need. Bit manipulation
code tends to be less "portable". Code is "portable" if with no programmer intervention it
compiles and runs correctly on different types of computers. The bitwise operations are
10
typically used with unsigned types. In particular, the shift operations are guaranteed to
shift 0 bits into the newly vacated positions when used on unsigned values.
~Bitwise Negation (unary) – flip 0 to 1 and 1 to 0 throughout
&Bitwise And
|Bitwise Or
^Bitwise Exclusive Or
>>Right Shift by right hand side (RHS) (divide by power of 2)
<
Do not confuse the Bitwise operators with the logical operators. The bitwise connectives
are one character wide (&, |) while the boolean connectives are two characters wide (&&,
||). The bitwise operators have higher precedence than the boolean operators. The
compiler will never help you out with a type error if you use & when you meant &&. As
far as the type checker is concerned, they are identical-- they both take and produce
integers since there is no distinct boolean type.
Other Assignment Operators
In addition to the plain = operator, C includes many shorthand operators which represents
variations on the basic =. For example "+=" adds the right hand side to the left hand side.
x = x + 10; can be reduced to x += 10;. This is most useful if x is a long
expression such as the following, and in some cases it may run a little faster.
person->relatives.mom.numChildren += 2;// increase children by 2
Here's the list of assignment shorthand operators...
+=, -=Increment or decrement by RHS
*=, /=Multiply or divide by RHS
%=Mod by RHS
>>=Bitwise right shift by RHS (divide by power of 2)
<<=Bitwise left shift RHS (multiply by power of 2)
&=, |=, ^=Bitwise and, or, xor by RHS
11
Section 2
Control StructuresCurly Braces {}
C uses curly braces ({}) to group multiple statements together. The statements execute in
order. Some languages let you declare variables on any line (C++). Other languages insist
that variables are declared only at the beginning of functions (Pascal). C takes the middle
road -- variables may be declared within the body of a function, but they must follow a
'{'. More modern languages like Java and C++ allow you to declare variables on any line,
which is handy.
If Statement
Both an if and an if-else are available in C. The can be any valid
expression. The parentheses around the expression are required, even if it is just a single
variable.
if () // simple form with no {}'s or else clause
if () {// simple form with {}'s to group statements
}
if () {// full then/else form
}
else {
}
Conditional Expression -or- The Ternary Operator
The conditional expression can be used as a shorthand for some if-else statements. The
general syntax of the conditional operator is:
? :
This is an expression, not a statement, so it represents a value. The operator works by
evaluating expression1. If it is true (non-zero), it evaluates and returns expression2 .
Otherwise, it evaluates and returns expression3.
The classic example of the ternary operator is to return the smaller of two variables.
Every once in a while, the
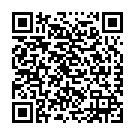
Continue reading on your phone by scaning this QR Code
Tip: The current page has been bookmarked automatically. If you wish to continue reading later, just open the
Dertz Homepage, and click on the 'continue reading' link at the bottom of the page.