and Ritchie. The thin book
which for years was the bible for all C programmers. Written by the original
designers of the language. The explanations are pretty short, so this book is better as a
reference than for beginners.
•http://cslibrary.stanford.edu/102/Pointers and Memory -- Much more detail
about local memory, pointers, reference parameters, and heap memory than in this
article, and memory is really the hardest part of C and C++.
•http://cslibrary.stanford.edu//103/Linked List Basics -- Once you understand the
basics of pointers and C, these problems are a good way to get more practice.
3
Section 1
Basic Types and OperatorsC provides a standard, minimal set of basic data types. Sometimes these are called
"primitive" types. More complex data structures can be built up from these basic types.
Integer Types
The "integral" types in C form a family of integer types. They all behave like integers and
can be mixed together and used in similar ways. The differences are due to the different
number of bits ("widths") used to implement each type -- the wider types can store a
greater ranges of values.
charASCII character -- at least 8 bits. Pronounced "car". As a practical matter
char is basically always a byte which is 8 bits which is enough to store a single
ASCII character. 8 bits provides a signed range of -128..127 or an unsigned range is
0..255. char is also required to be the "smallest addressable unit" for the machine --
each byte in memory has its own address.
shortSmall integer -- at least 16 bits which provides a signed range of
-32768..32767. Typical size is 16 bits. Not used so much.
intDefault integer -- at least 16 bits, with 32 bits being typical. Defined to be
the "most comfortable" size for the computer. If you do not really care about the
range for an integer variable, declare it int since that is likely to be an appropriate
size (16 or 32 bit) which works well for that machine.
longLarge integer -- at least 32 bits. Typical size is 32 bits which gives a signed
range of about -2 billion ..+2 billion. Some compilers support "long long" for 64 bit
ints.
The integer types can be preceded by the qualifier unsigned which disallows
representing negative numbers, but doubles the largest positive number representable. For
example, a 16 bit implementation of short can store numbers in the range
-32768..32767, while unsigned short can store 0..65535. You can think of pointers
as being a form of unsigned long on a machine with 4 byte pointers. In my opinion,
it's best to avoid using unsigned unless you really need to. It tends to cause more
misunderstandings and problems than it is worth.
Extra: Portability Problems
Instead of defining the exact sizes of the integer types, C defines lower bounds. This
makes it easier to implement C compilers on a wide range of hardware. Unfortunately it
occasionally leads to bugs where a program runs differently on a 16-bit-int machine than
it runs on a 32-bit-int machine. In particular, if you are designing a function that will be
implemented on several different machines, it is a good idea to use typedefs to set up
types like Int32 for 32 bit int and Int16 for 16 bit int. That way you can prototype a
function Foo(Int32) and be confident that the typedefs for each machine will be set so
that the function really takes exactly a 32 bit int. That way the code will behave the same
on all the different machines.
char Constants
A char constant is written with single quotes (') like 'A' or 'z'. The char constant 'A' is
really just a synonym for the ordinary integer value 65 which is the ASCII value for
4
uppercase 'A'. There are special case char constants, such as ' ' for tab, for characters
which are not convenient to type on a keyboard.
'A'uppercase 'A' character
'
'newline character
' 'tab character
'\0'the "null" character -- integer value 0 (different from the char digit '0')
'\
'the character with value 12 in octal, which is decimal 10
int Constants
Numbers in the source code such as 234 default to type int. They may be followed by
an 'L' (upper or lower case) to designate that the constant should be a long such as 42L.
An integer constant can be written with a leading 0x to indicate that it is expressed in
hexadecimal -- 0x10 is way of expressing the number 16. Similarly, a constant may be
written in octal by preceding it with "0" -- 012 is a way of expressing the number 10.
Type Combination and Promotion
The integral types may be mixed together in arithmetic expressions since they are all
basically just integers with variation in their width. For example, char and int can be
combined in arithmetic expressions such as ('b' + 5). How does the compiler deal
with the different widths present in such an expression? In such a case, the compiler
"promotes" the smaller type (char) to be the same size as the larger type (int) before
combining the values. Promotions are determined at compile time based purely on the
types of the values in the expressions. Promotions do not lose information -- they
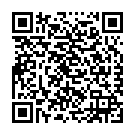
Continue reading on your phone by scaning this QR Code
Tip: The current page has been bookmarked automatically. If you wish to continue reading later, just open the
Dertz Homepage, and click on the 'continue reading' link at the bottom of the page.