zero
which is conventionally representing the NULL pointer. Here are some
examples:
char *pc; /* pointer to a char */
int *pv = &a; /* initialized to address of a */
short *ps = 0; /* initialized to NULL pointer */
A pointer can be declared with the special type void. Such a pointer is
handled by the compiler as a plain pointer regarding the assignement
operations, but the object pointed at by the pointer cannot be accessed
directly. Such a syntax is interesting when a pointer has to share differ-
ent types.
Arrays
3-4 Declarations © Copyright 2003 by COSMIC Software
3
Arrays
An array is declared with three parameters. The first one indicates that
the variable is an array, the second indicates how many elements are in
the array and the third is the type of one element. In order to match the
global syntax, the field is used to declare the type of one ele-
ment. The fact that the variable is an array and its dimension will be
indicated by adding the dimension written between square brackets
[10] after the name. The dimension is an integer constant which may
be omitted in some cases. An array initialization will be written by an
equal sign
= followed by a list of values placed between curly braces,
and separated by commas. Here are some examples:
char tab[10]; /* array of 10 chars */
int values[3] = {1, 2, 3};
An initialization list may be smaller than the dimension, but never
larger. If the list is smaller than the specified dimension, the missing
elements are filled with zeroes. If an initialization list is specified, the
dimension may be omitted. In that case, the compiler gives the array the
same length than the specified list:
int values[]={1, 2, 3};/* array of 3 elements */
An array of char elements can be initialized with a text string. written
as a sequence of characters enclose by double quotes characters:
char string[10] = “hello”;
The missing characters are filled with zeroes. Because a text string is
conventionally ended by a NULL character, the following declaration:
char string[] = “hello”;
defines an array of 6 characters, 5 for the word hello itself, plus one
for the ending NULL which will be appended by the compiler. Note
that if you write the following declaration:
char string[5] = “hello”;
Modifiers
© Copyright 2003 by COSMIC Software
Declarations 3-5 the compiler will declare an array of 5 characters, and will not com-
plain, or add any NULL character at the end. Any smaller dimension
will cause an error.
All these declarations may be applied recursively to themselves, thus
declaring pointers to pointers, array of arrays, arrays of pointers, point-
ers to arrays, and so on. To declare an array of 10 pointers to chars, we
write:
char *ptab[10];
But if we need to declare a pointer to an array of 10 chars, we should
write:
char *tabp[10];
Unfortunately, this is the same declaration for two distinct objects. The
mechanism as described above is not enough to allow all the possible
declarations without ambiguity. The C syntax for declaration uses prior-
ities to avoid ambiguities, and parentheses to modify the order of prior-
ities. The array indicators
[] have a greater priority than the pointer
indicator
*. Using this priority, the above example will always declare
an array of
10 pointers.
To declare a pointer to an array, parentheses have to be used to apply
first the pointer indicator to the name:
char (*tabp)[10];
Modifiers
A declaration may be completed by using a modifier. A modifier is
either of the keywords const and volatile or any of the space modifiers
accepted by the compiler. A space modifier is written with an at sign
@
followed by an identifier. The compiler accepts some predefined space
modifiers, available for all the target processors, plus several target spe-
cific space modifiers, available only for some target processors. The
COSMIC compiler provides three basic space modifiers for data
objects: @tiny, @near and @far. @tiny designates a memory space
for which a one byte address is needed. @near designates a memory
Modifiers
3-6 Declarations © Copyright 2003 by COSMIC Software
3
space for which a two byte address is needed. @far designates a mem-
ory space for which a four byte address is needed. The compilers are
provided with one or several different memory models implementing
various default behaviours, so if none of these space modifiers is speci-
fied, the selected memory model will enforce the proper one.
The const modifier means that the object to which it is applied is con-
stant. The compiler will reject any attempt to modify directly its value
by an assignment. A cross compiler goes further and may decide to
locate such a constant variable in the code area, which is normally writ-
ten in a PROM. A const object can be initialized only in its declaration.
When the initialised value of a const variable is known to the compiler,
because it is declared in the current file, an access to this variable will
be replaced by direct use of the initialised value. This behaviour can be
disabled by the -pnc compiler option if such an access must be imple-
mented by a memory access.
The volatile modifier means that the value of the object to
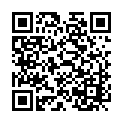
Continue reading on your phone by scaning this QR Code
Tip: The current page has been bookmarked automatically. If you wish to continue reading later, just open the
Dertz Homepage, and click on the 'continue reading' link at the bottom of the page.