This allows the same
syntax to be used for a function declaration, and also allows a pointer to
point at a function. A function is a piece of code performing a transfor-
mation. It receives information by its arguments, it transforms these
input and the global information, and then produces a result, either by
returning a value, or by updating some global variables. To help the
function in its work, it is allowed to have local variables which are
accessed only by that function and exist only when the function is
active. Arguments and local variables will generally be allocated on the
stack to allow recursivity. For some very small microprocessors, the
stack is not easily addressable and the compiler will not use the proces-
sor stack. It will either simulate a stack in memory if the application
needs to use recursivity, or will allocate these variables once for ever at
link time, reducing the entry/exit time and code load of each function,
but stopping any usage of recursivity.
When a function call is executed the arguments are copied onto the
stack before the function is called. After the call, the return value is cop-
ied to its destination and the arguments are removed from the stack. An
argument or a return value may be any of the C data objects. Objects are
copied, with the exception of arrays, whose address is copied and not
the full content. So passing an array as argument will only move a
pointer, but passing a structure as argument will copy the full content of
the structure on to the stack, whatever its size.
A C program may be seen as a collection of objects, each of these
objects being a variable or a function. An application has to start with a
specific function. The C environment defines the function called main
as the first one to be called when an application is starting. This is only
a convention and this may be changed by modifying the compiler envi-
ronment.
© Copyright 2003 by COSMIC Software
CHAPTER
Declarations 3-1
3
Declarations
An object declaration in C follows the global format:
;
This global format is shared by all the C objects including functions.
Each field differs depending on the object type.
is the storage class, and gives information about how the
object is allocated and where it is known and then accessible.
gives the basic type of the object and is generally completed by
the name information.
gives a name to the object and is generally an identifier. It
may be followed or preceded by additional information to declare com-
plex objects.
gives an initial value to the object. Depending on how
the object is allocated, it may be static information to be built by the
linker, or it may be some executable code to set up the variable when it
is created. This field is optional and may be omitted.
Each declaration is terminated by the semicolon character
;. To be
more convenient, a declaration may contain several occurrences of the
pair , separated by commas. Each variable
shares the same storage class and the same basic type.
Integers
3-2 Declarations © Copyright 2003 by COSMIC Software
3
Integers
An integer variable is declared using the following basic types:
char
short
or short int
int
long
or long int
These basic types may be prefixed by the keyword signed or unsigned.
The type unsigned int may be shortened by writing only unsigned.
Ty p e s short, int and long are signed by default. Type char is defaulted
to either signed char or unsigned char depending on the target proces-
sor. For most of the small microprocessors, a plain char is defaulted to
unsigned char.
A real variable is declared using the following basic types:
float
double
long double
In most of the cases, type long double is equivalent to type double. For
small microprocessors, all real types are mapped to type float.
For these numerical variables, an initialization is written by an equal
sign
= followed by a constant. Here are some examples:
char c;
short val = 1;
int a, b;
unsigned long l1 = 0, l2 = 3;
Bits
© Copyright 2003 by COSMIC Software
Declarations 3-3
Bits
A bit variable is declared with the following basic type:
_Bool
These variables can be initialised like a numerical type, but the assign-
ment rules do not match the integer rules as described in the next chap-
ter. Here are some examples:
_Bool ready;
_Bool led = 0;
It is not possible to declare a pointer to a bit variable, nor an array of bit
variables.
Pointers
A pointer is declared with two parameters. The first one indicates that
the variable is a pointer, and the second is the type of the pointed object.
In order to match the global syntax, the field is used to declare
the type of the pointed object. The fact that the variable is a pointer will
be indicated by prefixing the variable name with the character *.
Beware that declaring a pointer does not allocate memory for the
pointed object, but only for the pointer itself. The initialization field is
written as for a numerical variable, but the constant is an address con-
stant and not a numerical constant, except for the numerical value
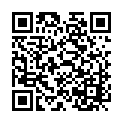
Continue reading on your phone by scaning this QR Code
Tip: The current page has been bookmarked automatically. If you wish to continue reading later, just open the
Dertz Homepage, and click on the 'continue reading' link at the bottom of the page.