list as a list of individual
declarations separated by commas between the parentheses.
int max(int a, int b)
The prototyped syntax offers extra features compared with the K&R
syntax. When a function is called with parameters passing, the compiler
will check that the number of arguments passed matches the number in
the declaration, and that each argument is passed with the expected
type. This means that the compiler will try to convert the actual argu-
ment into the expected type. If it is not possible, the compiler will pro-
duce an error message.
None of these checks are done when the function is declared with the
K&R syntax. If a function has no arguments, there should be no differ-
ence between the two syntaxes. To force the compiler to check that no
argument is passed, the keyword
void will replace the argument list.
int func(void)
The K&R syntax allows a variable number of arguments to be passed.
In order to keep this feature with the prototyped syntax, a special punc-
tuator has been introduced to tell the compiler that other arguments may
be specified, with unknown types. This is written by adding the
sequence
... as last argument.
int max(int a, int b, ...)
The compiler will check that there are at least two arguments, which
will be converted to int if they have a compatible type. It will not com-
plain if there are more than two arguments, and they will be passed
without explicit conversion.
Storage Class
© Copyright 2003 by COSMIC Software
Declarations 3-13 The prototyped syntax is preferred as it allows for more verification and
thus more safety. It is possible to force the COSMIC compiler to check
that a function has been properly prototyped by using the -pp or +strict
options.
The initialization of a function is the body of the function, meaning the
list of statements describing the function behaviour. These statements
are grouped in a block, and placed between curly braces
{}. There is no
equal sign as for the other initializations, and the ending semicolon is
not needed.
int max(int a, int b)
{
/* body of the function */
}
A function may have local variables which will be declared at the
beginning of the function block. In order to allow the recursivity, such
local variables and the arguments have to be located in an area allocated
dynamically. For most of the target microprocessors, this area is the
stack. This means that these local variables are not allocated in the same
way as the other variables.
Storage Class
It is possible in C to have a partial control over the way variables are
allocated. This is done with the field defining the storage
class. This information will also control the scope of the variable,
meaning the locations in the program where this variable is known and
then accessible. The storage class is one of the following keyword:
extern
static
auto
register
extern means that the object is defined somewhere else. It should not
be initialized, although such a practice is possible. The extern keyword
is merely ignored in that case. The definition may be incomplete. An
array may be defined with an unknown dimension, by just writing the
bracket pair without dimension inside.
Storage Class
3-14 Declarations © Copyright 2003 by COSMIC Software
3
extern int tab[];
A function may be declared with only the type of its arguments and of
its return value.
extern int max(int, int);
Note that for a function, the extern class is implied if no function body
is specified. Note also that if a complete declaration is written, useless
information (array dimension or argument names) is merely ignored.
The COSMIC compiler may in fact use a dimension array to optimize
the code needed to compute the address of an array element, so it may
be useful to keep the dimension even on an extern array declaration.
static means that the object is not accessible by all parts of the program.
If the object is declared outside a function, it is accessible only by the
functions of the same file. It has a file scope. If an object with the same
name is declared in another file, they will not describe the same mem-
ory location, and the compiler will not complain. If the object is
declared inside a function, it is accessible only by that function, just like
a local variable, but it is not allocated on the stack, meaning that the
variable keeps its value over the function calls. It has function scope.
auto means that the object is allocated dynamically, and this implies
that it is a local variable. This class cannot be applied to an object
declared outside a function. This is also the default class for an object
declared inside a function, so this keyword is most of the time omitted.
register means that the object is allocated if possible in a physical reg-
ister of the processor. This class cannot be applied to an object declared
outside a function. If the request cannot be satisfied, the class is ignored
and the variable is defaulted to an auto variable.
A register variable is more efficient, but generally only a few variables
can be mapped in registers. For most of the small microprocessors, all
the internal registers are used by the
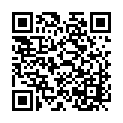
Continue reading on your phone by scaning this QR Code
Tip: The current page has been bookmarked automatically. If you wish to continue reading later, just open the
Dertz Homepage, and click on the 'continue reading' link at the bottom of the page.